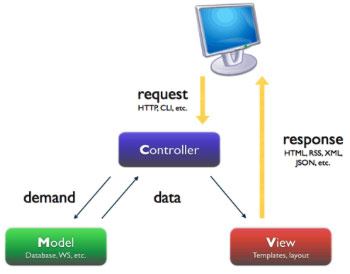
Model-View-Controller
The Model-View-Controller (MVC) is a design pattern used to design the user interface and activities of a software application. In other words, what does a web page or mobile application look like and how does it work.
How is the software designed so pictures, words, links and buttons show up, and what happens when someone clicks on a button or a hyperlink? The individual items of Model, View and Controller each serve a purpose; the Model is the business and related data and processes, the View is what is displayed to the end user and the Controller handles the events between what is displayed and how the business responds.
CakePHP framework MVC
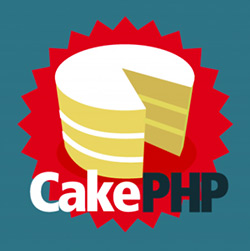
CONTROLLER
<?php
class BooksController extends AppController {
function list($category) {
$this->set('books', $this->Book->findAllByCategory($category));
}
function add() { ... ... }
function delete() { ... ... }
... ... } ?>
MODEL
?php
class Book extends AppModel {
}
?>
VIEW
<table> <tr> <th>Title</th> <th>Author</th> <th>Price</th> </tr>
<?php foreach ($books as $book): ?> <tr> <td> <?php echo $book['Book']['title']; ?> </td> <td> <?php echo $book['Book']['author']; ?> </td> <td> <?php echo $book['Book']['price']; ?> </td> </tr> <?php endforeach; ?>
</table>
Tier Architecture
The MVC implemented in a three-tier architecture
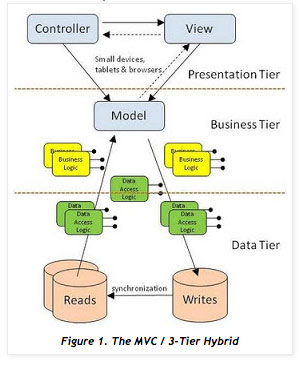
How do these two come together? From an MVC perspective the View and Controller exists in the presentation tier and the model spans the business and data tiers. From a three-tier perspective this means the Model is broken into modules, which are optimized based on their activity and for their ability to be reused and altered for new business opportunities. The Model will span the business and data tiers. The Controller and View exist in the presentation tier and this is also optimal for it allows the software developer to build, render and respond to user interfaces best designed for different devices (internet browsers and mobile devices).
n-tier architecture detailed
1) The Presentation Layer: Also called as the client layer is comprised of components that are dedicated to presenting the data to the user. For example: Windows/Web Forms and buttons, edit boxes, Text boxes, labels, grids, etc.
2) The Business Rules Layer: This layer encapsulates the Business rules or the business logic of the encapsulations. To have a separate layer for business logic is of a great advantage. This is because any changes in Business Rules can be easily handled in this layer. As long as the interface between the layers remains the same, any changes to the functionality/processing logic in this layer can be made without impacting the others.
3) The Data Access Layer: This layer comprises of components that help in accessing the Database. If used in the right way, this layer provides a level of abstraction for the database structures. Simply put, changes made to the database, tables, etc. do not affect the rest of the application because of the Data Access layer. The different application layers send the data requests to this layer and receive the response from this layer.
The database is not accessed directly from any other layer/component. Hence, the table names, field names are not hard coded anywhere else. This layer may also access any other services that may provide it with data, for instance, Active Directory, Services etc. Having this layer also provides an additional layer of security for the database. As the other layers do not need to know the database credentials, connect strings and so on.
4) The Database Layer: This layer is comprise of the Database Components such as DB Files, Tables, Views, etc. The Actual database could be created using SQL Server, Oracle, Flat files, etc.